Procedural Generation & Spatial Optimization in C++ with Raylib
Technology Stack
- Language: C++
- Library: Raylib (including core, Raymath, rlgl modules)
Sometimes it's easy to dive in Unreal Engine 5 ecosystem and program in C++. I felt that the hardest bit of that experience, is the Unreal Engine documentation.
While UE5 provides powerful abstractions, I felt that exploring graphics programming and core system implementation with fewer engine layers would be a valuable exercise. For this, I chose Raylib - a fantastic library that provides helpful abstractions for OpenGL and low-level functionalities, while still keeping me close to the core C++ development process.
High-Level Overview
SpaceCPP is a 3D tech demo featuring a procedurally generated asteroid field, a first-person camera, basic particle effects, and UI elements. The entire project was built from scratch using C++ and the lightweight Raylib library.
The primary motivation behind this project was to deepen my C++ programming skills outside the context of a large, established game engine like Unreal Engine 5. It served as a practical exercise to explore and implement several key game development concepts directly:
- Procedural mesh generation: Creating unique 3D assets algorithmically.
- Spatial partitioning: Implementing a Uniform Grid to optimize collision detection performance.
- Core system implementation: Building components like a custom camera controller and a particle system in C++.
- View the Code on GitHub: https://github.com/alessandrobelli/SpaceRaylibCpp
Key Technical Features & Implementation Deep Dive
Procedural Asteroid Generation
Manually creating hundreds or thousands of unique asteroid models would be extremely time-consuming. To address this, a C++ function (GenerateAsteroidMesh
in asteroid_field.cpp
) was implemented to generate varied and natural-looking asteroid shapes programmatically.
The process starts by creating a base sphere mesh using Raylib's GenMeshSphere
, incorporating randomized detail levels (rings and slices) for initial variation. The function then accesses the raw vertex data of this mesh via a float*
pointer and iterates through each vertex. For each one, it calculates a random offset direction (usually outwards from the center) and magnitude, influenced by the asteroid's base radius and an overall irregularity
parameter. This offset is applied to the vertex's original position using Raymath vector functions (Vector3Add
, Vector3Scale
, Vector3Normalize
), and the modified position is written directly back into the mesh's vertex buffer, resulting in a unique, irregular mesh.
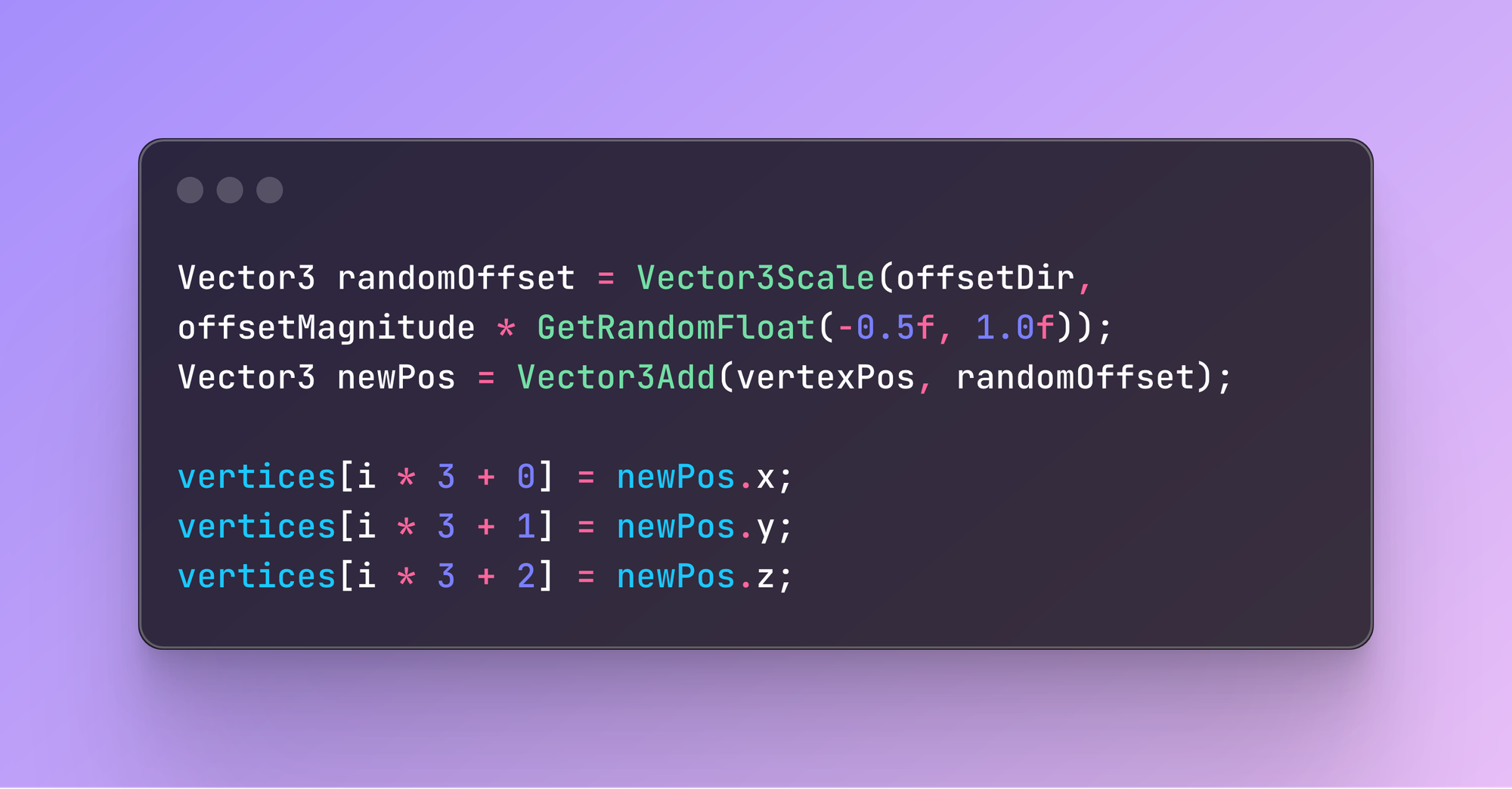
Uniform Grid for Collision Optimization
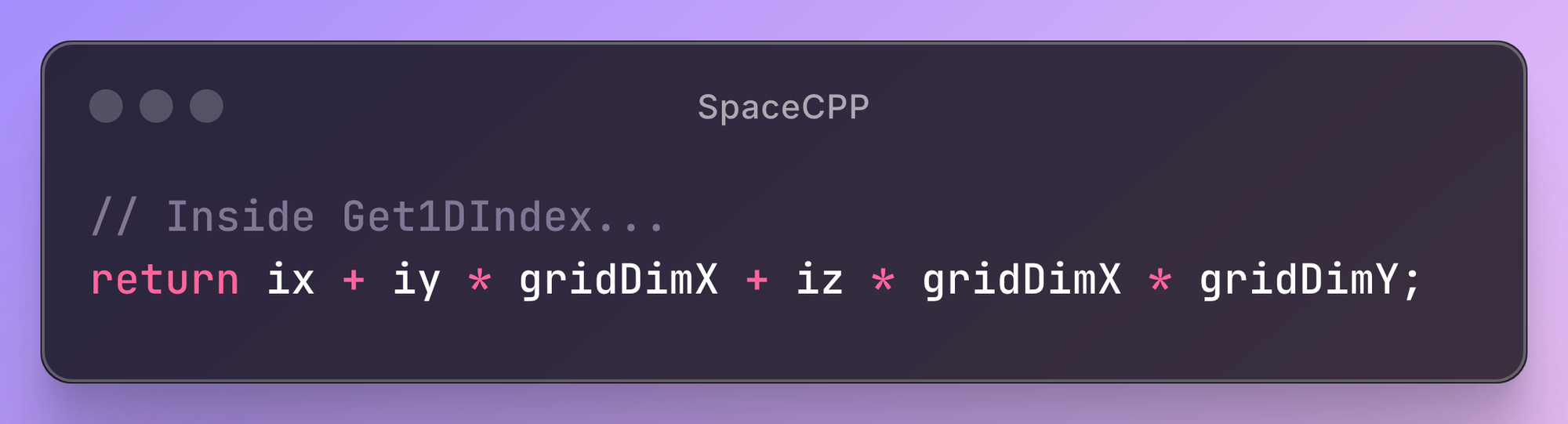
Checking every asteroid for collision against the player or handling raycasts naively would be computationally expensive given the potential number of asteroids. To optimize this, a Uniform Grid spatial partitioning structure (UniformGrid
class) was implemented in C++.
The system divides the relevant world space into a 3D grid of equal-sized cells. During initialization, each asteroid's index is stored in a list associated with every grid cell its bounding box overlaps (Add
and BuildInstanced
methods handle this). The grid data is stored in a std::vector
where each element represents a cell and holds another std::vector<int>
containing the indices of overlapping asteroids.
When performing checks:
- Point-based queries (like checking the player's position) only need to examine the objects listed in the cell containing the point and its immediate neighbors (
Query
method). - Raycasts use a fast voxel traversal algorithm (
QueryRay
method) to identify only the cells the ray passes through. This approach drastically reduces the number of asteroids that need detailed collision or intersection tests each frame.
Custom C++ Systems
Beyond these specific algorithms, several core components were built from scratch in C++:
- First-Person Camera (
CustomCamera
): A class manages the 3D camera, handling mouse input for orientation (yaw/pitch) and keyboard input for movement (WASD/Space/Ctrl) relative to where the camera is facing. - Particle System: A simple particle engine uses a fixed-size object pool. This allows for efficient emission and rendering of effects (like explosions) by recycling inactive particle instances rather than performing frequent memory allocations.
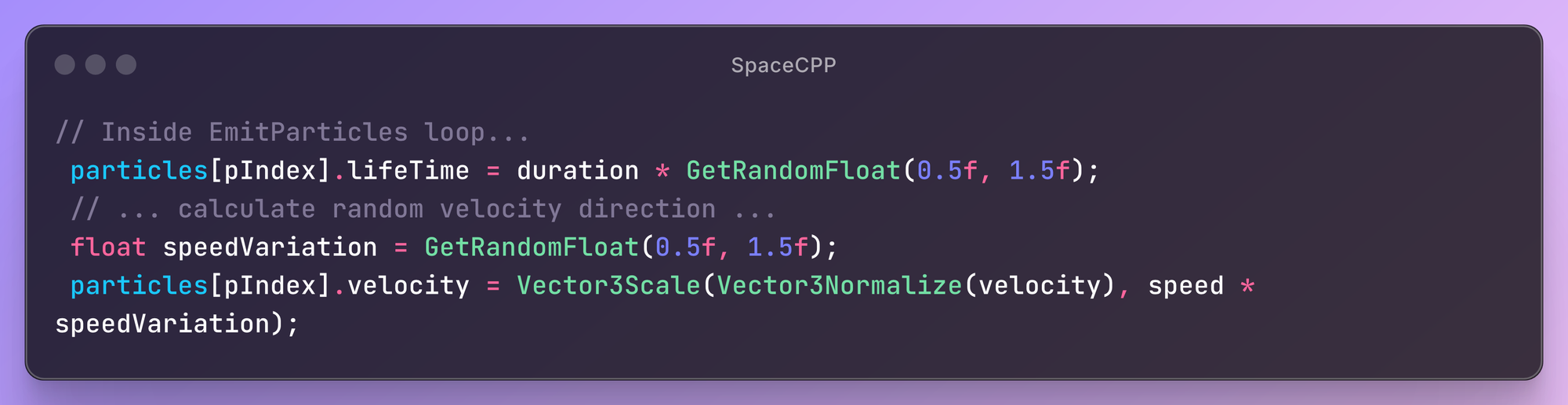
Challenges & Learning Outcomes
Developing the uniform grid, particularly the ray traversal logic (QueryRay
), presented an interesting algorithmic challenge that required careful implementation and debugging. Tuning the procedural generation parameters in GenerateAsteroidMesh
to get consistently varied but natural-looking results also involved iterative refinement.
Building this project provided valuable experience with graphics programming concepts, implementing spatial partitioning techniques for performance, and managing the structure of a small 3D application in C++.